Stepping
AndroidPlot uses the concept of "stepping" to calculate where and how many ticks are drawn. An XYPlot has a domain and a range axis, each of which has an independent stepping algorithm associated with it. These algorithms are set using XYPlot.setDomainStep() and XYPlot.setRangeStep() methods. Let's take a look at the arguments for these methods:
- XYStepMode mode : The XYStepMode to use.
- double value : value used in conjunction with mode.
Next, let's see how each XYStepMode works:
XYStepMode.SUBDIVIDE
This is the default XYStepMode used for each axis. The default value assigned to this mode is 10. Simply put, this tells XYPlot to evenly subdivide the plot using 10 ticks. Here's how the Quickstart project looks by default:
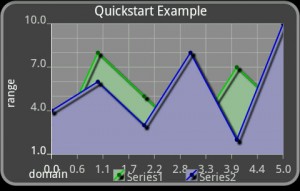
As you can see, there are 10 domain ticks and 10 range ticks. If we modify the code like this:
mySimpleXYPlot.setDomainStep(XYStepMode.SUBDIVIDE, 5);
We then get the following:
XYStepType.INCREMENT_BY_VALUE
This mode creates a tick for every multiple of value above and below the origin. Let's modify the quickstart example like this:
mySimpleXYPlot.setDomainStep(XYStepMode.INCREMENT_BY_VAL, 1);
This tells XYPlot to draw a tick for every visible domain value (x) where the modulus of x minus domainOrigin is zero, or to put it more simply, draw a tick at every increment of 1 starting from domainOrigin. Our plot now looks like this:
Notice how each tick on the domain exactly coincides with a point that has been plotted. If we wanted to further subdivide the domain without sacrificing this alignment, we can reduce the increment to any value where 1 % newval == 0. Here's what 0.5 looks like:
mySimpleXYPlot.setDomainStep(XYStepMode.INCREMENT_BY_VAL, 0.5);
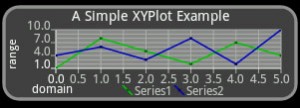
XYStepType.INCREMENT_BY_PIXELS
This mode is very similar to INCREMENT_BY_VAL but instead of stepping by values, each tick is spaced out by a user defined number of pixels.
Formatting
Before Domain and Range values are drawn onto an XYPlot, they are first formatted using an associated instance of
java.text.Format. The active Format instance may be accessed via these XYPlot methods:
- Format getRangeValueFormat()
- setRangeValueFormat(Format)
- Format getDomainValueFormat()
- setDomainValueFormat(Format)
Any class extending Format, including user-created classes can be used to format the domain/range values. By default, AndroidPlot uses an instance of
java.text.NumberFormat.
Building on the previous examples, lets modify the quickstart code to use a custom domain Format:
// draw a domain line for every element plotted on the domain:
mySimpleXYPlot.setDomainStep(XYStepMode.INCREMENT_BY_VAL, 1);
// get rid of the decimal place on the display:
mySimpleXYPlot.setDomainValueFormat(new DecimalFormat("#"));
The second statement is new. If you go back up to the previous examples you will notice that the domain value labels are printed with decimal places regardless of whether or not there is a fractional part to the number. With our newly assigned DecimalFormat, we now get the following:
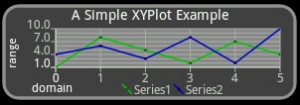
It looks pretty simple, but it's actually quite powerful; you can use Format instances to display scientific notation, dates and times, states in a state machine or pretty much anything else you can think of.